mcuApi.h File Reference
#include <boost/function.hpp>
#include <boost/system/error_code.hpp>
#include <boost/shared_ptr.hpp>
#include <list>
#include "mcuTypes.h"
#include "firmware.h"
Include dependency graph for mcuApi.h:
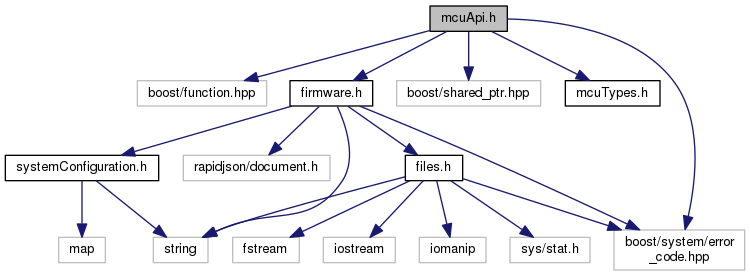
This graph shows which files directly or indirectly include this file:
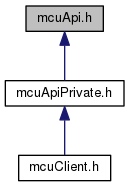
Go to the source code of this file.
Classes | |
struct | LedsConfig |
struct | GpioConfig |
GPIO Struct which contains the response received from the service. More... | |
struct | I2CValue |
I2C Struct which contains the response received from the service. More... | |
struct | UpdateConfig |
Update struct which contains the response received from the service. More... | |
struct | PowerInformationConfig |
PowerInformation struct which contains the response received from the service. More... | |
struct | PowerStateConfig |
PowerInformation struct which contains the response received from the service. More... | |
struct | TimersConfig |
Timers struct which contains the response received from the service. More... | |
struct | GUIDConfig |
GUID struct which contains the response received from the service. More... | |
struct | GUIDConfigList |
GUID list struct which contains the response received from the service. More... | |
struct | WorkingTimeConfig |
Working Time struct which contains the response received from the service. More... | |
struct | MCUStatus |
MCU Status struct which contains the response received from the service. More... | |
struct | BatteryProtectionConfig |
Battery Protection Config struct which contains the response received from the service. More... | |
struct | DateConfig |
DateConfig struct which contains the response received from the service. More... | |
class | Mcu |
Class which interfaces with the API logic. More... | |
Namespaces | |
dpyMcu | |
Deepsy Mcu namespace that includes the different enums, structs or method signatures that should be used. | |
Typedefs | |
typedef boost::function< void(const bool &available)> | service_availability_handler |
Prototype of the handler function used to monitor service availability. More... | |
typedef boost::function< void(boost::system::error_code error_code, dpyMcu::PowerInformationConfig &)> | power_mng_handler_function |
Prototype of the handler function for obtaining the Power Information. More... | |
typedef boost::function< void(boost::system::error_code error_code, dpyMcu::LedsConfig &)> | leds_handler_function |
Prototype of the handler function for obtaining the LEDs configuration. More... | |
typedef boost::function< void(boost::system::error_code error_code, dpyMcu::PowerStateConfig &)> | power_states_handler_function |
Prototype of the handler function for obtaining the Power State Configuration. More... | |
typedef boost::function< void(boost::system::error_code error_code, dpyMcu::TimersConfig &)> | timers_handler_function |
Prototype of the handler function for obtaining the Timers Configuration. More... | |
typedef boost::function< void(boost::system::error_code error_code, dpyMcu::UpdateConfig &)> | update_firmware_handler_function |
Prototype of the handler function for obtaining the updated configuration. More... | |
typedef boost::function< void(boost::system::error_code error_code, dpyMcu::GUIDConfigList &)> | guid_handler_function |
Prototype of the handler function for obtaining the GUIDs. More... | |
typedef boost::function< void(boost::system::error_code error_code, char &, char &)> | eqvar_handler_function |
Prototype of the handler function for obtaining the GUIDs. More... | |
typedef boost::function< void(boost::system::error_code error_code, dpyMcu::WorkingTimeConfig &)> | working_time_handler_function |
Prototype of the handler function for obtaining the Working Time Values. More... | |
typedef boost::function< void(boost::system::error_code error_code, dpyMcu::MCUStatus &)> | mcu_status_handler_function |
Prototype of the handler function for obtaining the MCU Status. More... | |
typedef boost::function< void(boost::system::error_code error_code, dpyMcu::BatteryProtectionConfig &)> | battery_protection_handler_function |
Prototype of the handler function for obtaining the Battery Protection Configuration. More... | |
typedef boost::function< void(boost::system::error_code error_code, dpyMcu::DateConfig &)> | rtc_date_handler_function |
Prototype of the handler function for obtaining the RTC Date Configuration. More... | |
typedef boost::function< void(boost::system::error_code error_code, dpyMcu::GpioConfig &)> | gpio_handler_function |
Prototype of the handler function for obtaining the GPIO Configuration. More... | |
typedef boost::function< void(boost::system::error_code error_code, dpyMcu::GpiosAvailable &)> | gpios_events_handler_function |
Prototype of the handler function for obtaining the GPIO Events. More... | |
typedef boost::function< void(boost::system::error_code error_code, dpyMcu::I2CValue &)> | i2c_handler_function |
Prototype of the handler function for obtaining the I2C information. More... | |
typedef boost::function< void(boost::system::error_code error_code, const dpyFirmware::FirmwareVersionInfo &fw_version)> | version_handler_function |
Prototype of the handler function for obtaining the fw version. More... | |
typedef boost::function< void(boost::system::error_code error_code, dpyMcu::CanStatusEvent &)> | can_status_handler_function |
Prototype of the handler function for obtaining the CAN Status Information. More... | |
typedef boost::function< void(boost::system::error_code error_code)> | test_misc_handler_function |
Prototype of the handler function for obtaining the current MISC status. More... | |
typedef boost::function< void(boost::system::error_code error_code)> | set_power_button_state_handler_function |
Prototype of the handler function for obtaining the result of power button. More... | |
typedef boost::function< void(boost::system::error_code error_code, bool enabled)> | get_power_button_state_handler_function |
Prototype of the handler function for obtaining the result of power button. More... | |
typedef boost::function< void(boost::system::error_code error_code)> | result_handler_function |
Prototype of the handler function for obtaining the result of the operations status. More... | |
Enumerations | |
MCU information | |
This enumerations give easy access to the main parameters that describe the MCU parameters in a given moment | |
enum | LedsColor { RED = 1, BLUE = 2, GREEN = 3, MAGENTA = 4, YELLOW = 5, CYAN = 6, UNDEFINED = 7 } |
Possible color to be set. More... | |
enum | PowerStates { N_A = 1, POWER_ON = 2, REBOOT = 3, STANDBY = 4, HALT = 5, ABORT = 6, CRITICAL_BATTERY = 7 } |
Possible power state to be set. More... | |
enum | GpiosAvailable { GPI1 = 1, GPI2 = 2, GPI3 = 3, GPI4 = 4, GPI5 = 5, GPI6 = 6, GPI7 = 7, GPI8 = 8, BUTTON1 = 9, BUTTON2 = 10, BUTTON3 = 11, BUTTON4 = 12, BUTTON5 = 13, BUTTON6 = 14, BUTTON7 = 15, BUTTON8 = 16, POWER_BUTTON = 17, ODO = 18, ODO_REDU = 19, IGN = 20, GPO1 = 21, GPO2 = 22, GPO3 = 23, GPO4 = 24, GPO5 = 25, GPO6 = 26, GPO7 = 27, GPO8 = 28 } |
Possible color to be set. More... | |
enum | Result { OK = 0, ERROR = 1 } |
Possible received result. More... | |
Detailed Description
- Copyright
- GMV, S.A. 2017. Property of GMV,S.A.; all rights reserved