Mcu Class Reference
Class which interfaces with the API logic.
#include <mcuApi.h>
Inheritance diagram for Mcu:
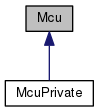
Public Member Functions | |
Mcu (std::string ip="127.0.0.1") | |
Constructor of the class. More... | |
virtual | ~Mcu () |
Destructor of the class. | |
bool | isAlive () |
Method to check if MCU Service is Alive. More... | |
void | monitorServiceAvailability_S (dpyMcu::service_availability_handler handler) |
void | monitorServiceAvailability_U () |
void | asyncGetLedsConfig (dpyMcu::leds_handler_function handler) |
Method which requests the LED color state. More... | |
void | asyncSetLedsConfig (dpyMcu::leds_handler_function handler, dpyMcu::LedsColor color, std::uint32_t period, std::uint32_t duty_cycle) |
Method which requests to change the LED color state. More... | |
void | asyncSetBatteryProtectionConfig (dpyMcu::battery_protection_handler_function handler, float idle_voltage, float voltage_percentage_threshold, float hysteresis_percentage, std::uint32_t grace_period_minutes) |
Method which requests to set battery protection configuration values. More... | |
void | asyncGetBatteryProtectionConfig (dpyMcu::battery_protection_handler_function handler) |
Method which requests to get battery protection configuration values. More... | |
void | asyncGetRtcDateConfig (dpyMcu::rtc_date_handler_function handler) |
Method which requests to get RTC date configuration values. More... | |
boost::system::error_code | getRtcDateConfig (dpyMcu::DateConfig &config) |
Method which requests to get RTC date configuration values. More... | |
void | asyncGetTimersConfig (dpyMcu::timers_handler_function handler) |
Method which requests one message with Standby and Halt Timers. More... | |
void | asyncUpdateMCU (dpyMcu::update_firmware_handler_function handler) |
Method which updates the firmware of the MCU (the new image must be in the right PATH of the system) More... | |
void | asyncGetGUIDValues (dpyMcu::guid_handler_function handler) |
Method which requests one message with Part Number, Serial Number and CIDL of the equipment. More... | |
virtual boost::system::error_code | getGUIDValues (dpyMcu::GUIDConfigList &guid_list) |
Method which requests to get GUID values. More... | |
void | asyncGetEQVar (dpyMcu::eqvar_handler_function handler) |
Method which requests to get EQ/Var values. More... | |
virtual boost::system::error_code | getEQVar (char &eq_id, char &var_id) |
Method which requests to get EQ/Var values. More... | |
void | asyncGetWorkingTimeValues (dpyMcu::working_time_handler_function handler) |
Method which requests one message with the Power-On, Standby and Halt States working hours. More... | |
void | asyncGetMCUStatus (dpyMcu::mcu_status_handler_function handler) |
Method which gets one message indicating hardware and software exceptions coming from MCU. More... | |
void | getPowerInformation_S (dpyMcu::power_mng_handler_function handler) |
Method which receives one message with Power information coming from the service periodically. More... | |
void | asyncGetPowerInformation (dpyMcu::power_mng_handler_function handler) |
Method which requests one message with Power information coming from the service periodically. More... | |
void | asyncGetGpioValue (dpyMcu::gpio_handler_function handler, std::uint32_t gpio_number) |
Method which requests one message with GPIO information. More... | |
void | asyncSetGpioValue (dpyMcu::gpio_handler_function handler, std::uint32_t gpio_number, bool gpio_value) |
Method which requests to set a GPIO pin with a value. More... | |
void | asyncGetGpiosEvents (dpyMcu::gpios_events_handler_function handler) |
Method which receives one message with Gpios events coming from the service. More... | |
void | asyncGetI2CValue (dpyMcu::i2c_handler_function handler, unsigned char busid, unsigned char deviceid, std::list< unsigned char > address, int lenght) |
Method which requests one message with I2C information. More... | |
void | asyncSetI2CValue (dpyMcu::i2c_handler_function handler, unsigned char busid, unsigned char deviceid, std::list< unsigned char > address, std::list< unsigned char > values) |
Method which requests to set a I2C address pin with a value. More... | |
void | asyncGetVersion (dpyMcu::version_handler_function handler, dpyFirmware::Rfs rfs) |
Method which requests the firware version of the MCU. More... | |
void | getPowerInformation_U () |
Method to stop receiving Power information coming from the service periodically. | |
boost::system::error_code | setI2CValue (unsigned char busid, unsigned char deviceid, std::list< unsigned char > address, std::list< unsigned char > values, dpyMcu::I2CValue &result) |
(Sync) Method which requests to set a I2C address pin with a value More... | |
boost::system::error_code | getI2CValue (unsigned char busid, unsigned char deviceid, std::list< unsigned char > address, int length, dpyMcu::I2CValue &result) |
(Sync) Method which requests one message with I2C information More... | |
void | asyncGetCanStatus (dpyMcu::can_status_handler_function handler) |
Method to get the current CAN Bus status. More... | |
Protected Attributes | |
boost::shared_ptr< McuClient > | mClient |
Reference to an Client Object. | |
Constructor & Destructor Documentation
|
explicit |
- Parameters
-
ip IP address to be binded to the App
Member Function Documentation
void asyncGetBatteryProtectionConfig | ( | dpyMcu::battery_protection_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetCanStatus | ( | dpyMcu::can_status_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetEQVar | ( | dpyMcu::eqvar_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetGpiosEvents | ( | dpyMcu::gpios_events_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetGpioValue | ( | dpyMcu::gpio_handler_function | handler, |
std::uint32_t | gpio_number | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service gpio_number GPIO Number (Possible values are 1, 2, 3 or 4)
void asyncGetGUIDValues | ( | dpyMcu::guid_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetI2CValue | ( | dpyMcu::i2c_handler_function | handler, |
unsigned char | busid, | ||
unsigned char | deviceid, | ||
std::list< unsigned char > | address, | ||
int | lenght | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service busid bus address deviceid device address address memory address lenght number of bytes to read
void asyncGetLedsConfig | ( | dpyMcu::leds_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetMCUStatus | ( | dpyMcu::mcu_status_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetPowerInformation | ( | dpyMcu::power_mng_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetRtcDateConfig | ( | dpyMcu::rtc_date_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetTimersConfig | ( | dpyMcu::timers_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetVersion | ( | dpyMcu::version_handler_function | handler, |
dpyFirmware::Rfs | rfs | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service rfs chosen to obtain the fw version installed (Possible values are "current" or "alternative")
void asyncGetWorkingTimeValues | ( | dpyMcu::working_time_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncSetBatteryProtectionConfig | ( | dpyMcu::battery_protection_handler_function | handler, |
float | idle_voltage, | ||
float | voltage_percentage_threshold, | ||
float | hysteresis_percentage, | ||
std::uint32_t | grace_period_minutes | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service idle_voltage idle voltage identified by ignition position off, engine off and stationary vehicle range: 0 - 40). Setting this value to 0 disables battery protection mode voltage_percentage_threshold voltage percentage calculated over idle voltage to set voltage battery protection mode threshold (range: 80 - 100) hysteresis_percentage hysteresis percentage calculated over idle voltage to enter and exit in battery protection mode (range: 0 - 100) grace_period_minutes grace period in minutes used to enter in halt mode irrevocably (range: 1 - 10)
- Remarks
- NOTE: With these values a low and a high threshold will be used to detect battery protection according next formula: – low_voltage_threshold = (idle_voltage * voltage_percentage / 100) - (idle_voltage * hysteresis_percentage / 100) – high_voltage_threshold = (idle_voltage * voltage_percentage / 100) + (idle_voltage * hysteresis_percentage / 100)
void asyncSetGpioValue | ( | dpyMcu::gpio_handler_function | handler, |
std::uint32_t | gpio_number, | ||
bool | gpio_value | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service gpio_number GPIO number (Possible values are 1, 2, 3 or 4) gpio_value GPIO value (Possible values are 1 or 0)
void asyncSetI2CValue | ( | dpyMcu::i2c_handler_function | handler, |
unsigned char | busid, | ||
unsigned char | deviceid, | ||
std::list< unsigned char > | address, | ||
std::list< unsigned char > | values | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service busid bus address deviceid device address address memory address. It's just ONE value. If you provide several values they will be concatenated to generate only ONE address. E.g. If you provide 0x10 0x20 0x30, it will produce a 0x102030 final address values values to be set
void asyncSetLedsConfig | ( | dpyMcu::leds_handler_function | handler, |
dpyMcu::LedsColor | color, | ||
std::uint32_t | period, | ||
std::uint32_t | duty_cycle | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service color to be set period periodic time to be set in seconds (range: 0 - 40) duty_cycle duty cycle to be set in percentage (range: 1 - 100)
void asyncUpdateMCU | ( | dpyMcu::update_firmware_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
|
virtual |
- Parameters
-
eq_id equipment identifier var_id varian identifier
- Returns
- error code
|
virtual |
- Parameters
-
guid_list struct containing Part Number, Serial Number and CIDL of the equipment
- Returns
- error code
boost::system::error_code getI2CValue | ( | unsigned char | busid, |
unsigned char | deviceid, | ||
std::list< unsigned char > | address, | ||
int | length, | ||
dpyMcu::I2CValue & | result | ||
) |
- Parameters
-
busid bus address deviceid device address address memory address length number of bytes to read result I2C structure to be filled with the response
- Returns
- error code
void getPowerInformation_S | ( | dpyMcu::power_mng_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
boost::system::error_code getRtcDateConfig | ( | dpyMcu::DateConfig & | config | ) |
- Parameters
-
config RTC date config
- Returns
- error code
bool isAlive | ( | ) |
TESTING : define this flag to fake/mock DPY API methods to be able to unit test classes which use DPY APIs.
- Returns
- Boolean indicating if MCU is alive
boost::system::error_code setI2CValue | ( | unsigned char | busid, |
unsigned char | deviceid, | ||
std::list< unsigned char > | address, | ||
std::list< unsigned char > | values, | ||
dpyMcu::I2CValue & | result | ||
) |
- Parameters
-
busid bus address deviceid device address address memory address. It's just ONE value. If you provide several values they will be concatenated to generate only ONE address. E.g. If you provide 0x10 0x20 0x30, it will produce a 0x102030 final address values values to be set result I2C structure to be filled with the response
- Returns
- error code