ModemManager Class Reference
Allows to interact with Modem Service.
#include <modemApi.h>
Inheritance diagram for ModemManager:
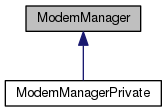
Public Member Functions | |
ModemManager (std::string ip="127.0.0.1") | |
Constructor of the class. More... | |
~ModemManager () | |
Destructor of the class. | |
bool | isAlive () |
Method to check if Modem Service is alive. More... | |
void | monitorServiceAvailability_S (dpyModem::service_availability_handler_function handler) |
Monitor Modem service availability. More... | |
void | monitorServiceAvailability_U () |
Stop monitoring Modem service availability. | |
void | asyncGetModems (dpyModem::modem_list_handler_function handler) |
Method which requests available modems. More... | |
void | asyncGetModemInfo (const std::string &modemid, dpyModem::modem_info_handler_function handler) |
Method which requests modem information. More... | |
void | asyncGetModemStatus (const std::string &modemid, dpyModem::modem_status_handler_function handler) |
Method which requests modem status. More... | |
void | getModemStatusEvents_S (dpyModem::modem_status_handler_function handler) |
Establish the handler that the subscriber will call when a new modem status (coming from any modem) is received. More... | |
void | getModemListEvents_S (dpyModem::modem_list_event_handler_function handler) |
Subscribes to modem list events. More... | |
void | getModemWarningEvents_S (dpyModem::modem_warning_event_handler_function handler) |
Subscribes to modem warning events. More... | |
void | asyncRequestModemOperation (const std::string &modemid, const dpyModem::ModemOperation &operation, dpyModem::result_handler_function handler) |
Query a specific modem to perform a specific operation. More... | |
void | asyncIsDataConnectionEnabled (const std::string &modemid, dpyModem::modem_data_connection_status_handler_function handler) |
Query if a specific modem data connection functionalities are enabled or not. More... | |
void | asyncForceCustomApn (dpyModem::result_handler_function handler, const bool &force=false, const dpyModem::AccessPointNetwork &custom_apn={}) |
Allows to select a specific APN manually ignoring the APN list (disabled by default). By default and, in order to make modem data connection easier for the user, the modem service selects the APN automatically by using a APN list. Making use of this method the user may ignore the list to make sure the custom_apn APN is used. More... | |
void | asyncGetInterfaceStatistics (const std::string &modemid, dpyModem::interface_statistics_function_handler) |
Allows to obtain different statistics of the interface related to transmission and reception of packages. More... | |
void | asyncForceNetworkMode (const std::string &modemid, dpyModem::NetworkMode mode, dpyModem::result_handler_function handler) |
Force a modem to work under a specific Network Mode (2G,3G,4G,...) More... | |
void | asyncGetForcedAPN (dpyModem::forced_apn_handler_function handler) |
Obtain the Forced APN configured on the device. If there is no forced APN, it returns an empty one. More... | |
Sim related methods | |
void | asyncGetModemSims (const std::string &modemid, dpyModem::sim_list_handler_function handler) |
Method which requests sims associated with a specific modem. More... | |
void | asyncGetActiveSims (const std::string &modemid, dpyModem::sim_list_handler_function handler) |
Method which requests sims being in use for a specific modem. More... | |
void | asyncSwitchCurrentSimCard (const std::string &modemid, dpyModem::result_handler_function handler) |
Change current Sim card being used by the specified modem, and start using alternative sim. More... | |
void | getCurrentSimSlot (const std::string &modemid, dpyModem::sim_slot_handler_function handler) |
Get current Sim card slot being used by the specified modem. More... | |
void | getSimListEvents_S (dpyModem::sim_list_event_handler_function handler) |
Subscribes to sim list events. More... | |
void | getSimStatusEvents_S (dpyModem::simStatus_handler_function handler) |
Subscribes to sim status events. More... | |
void | getSimStatusEvents_U () |
Method which requests sims associated with a specific modem. More... | |
void | asyncGetSimInfo (const std::string &simid, dpyModem::sim_info_handler_function handler) |
Method which requests modem information. More... | |
void | asyncGetSimStatus (const std::string &simid, dpyModem::simStatus_handler_function handler) |
Method which requests sim status. More... | |
Apn related methods | |
These methods allow to perform different APN operations. | |
void | asyncGetApnByImsi (const std::string &imsi, dpyModem::get_apn_by_imsi_handler_function handler) |
Obtain the APN that corresponds to the specified imsi as it would be obtained with the APN list. More... | |
void | asyncAddNewApn (const std::string &imsi, const dpyModem::AccessPointNetwork &apn, dpyModem::result_handler_function handler) |
Add a new APN to the APN list being used by the Modem when performing Modem data communications. More... | |
void | asyncDeleteApnByImsi (const std::string &imsi, dpyModem::result_handler_function handler) |
Delete a particular APN from the APN list by specifying the IMSI. More... | |
void | asyncModifyApnByImsi (const std::string ¤t_imsi, const dpyModem::AccessPointNetwork &new_apn, dpyModem::result_handler_function handler) |
Modify an existing APN (associated with a specific imsi) in the APN list. More... | |
void | asyncForceCustomApn (const std::string &imsi, dpyModem::result_handler_function handler, const bool &force=false, const dpyModem::AccessPointNetwork &custom_apn={}) |
Allows to select a specific APN manually ignoring the APN list (disabled by default). By default and, in order to make modem data connection easier for the user, the modem service selects the APN automatically by using a APN list. Making use of this method the user may ignore the list to make sure the custom_apn APN is used. More... | |
Protected Attributes | |
boost::shared_ptr< ModemClient > | mClient |
Reference to an Client Object. | |
Constructor & Destructor Documentation
|
explicit |
- Parameters
-
ip IP address to be binded to the App
Member Function Documentation
void asyncAddNewApn | ( | const std::string & | imsi, |
const dpyModem::AccessPointNetwork & | apn, | ||
dpyModem::result_handler_function | handler | ||
) |
- Parameters
-
imsi the value that will be associated with the apn apn the access point network structure that will be added to the list handler callback function
In order to understand the reason of the error code returned in the handler, please attend the following table:
ec (DPY_ERROR) | Result meaning |
---|---|
DPY_OK | The request was processed correctly |
DPY_DUPLICATE | The APN entry will not be added. The same APN entry already exists in the list and, therefore, it would be duplicated in case its added |
void asyncDeleteApnByImsi | ( | const std::string & | imsi, |
dpyModem::result_handler_function | handler | ||
) |
- Parameters
-
imsi the value of the imsi whose apn information will be removed handler callback function
In order to understand the reason of the error code returned in the handler, please attend the following table:
ec (DPY_ERROR) | Result meaning |
---|---|
DPY_OK | The request was processed correctly |
DPY_NOT_FOUND | The APN could not be deleted |
DPY_NOT_PERMITTED | The APN to delete is being used at the moment and therefore it can't be deleted |
void asyncForceCustomApn | ( | const std::string & | imsi, |
dpyModem::result_handler_function | handler, | ||
const bool & | force = false , |
||
const dpyModem::AccessPointNetwork & | custom_apn = {} |
||
) |
- Parameters
-
imsi the value of the imsi whose apn wants to be forced handler callback function force flag that indicates if a manual selection is desired of not, true if desired , false if the APN is selected from the list (default) custom_apn the APN that wants to be set, in case force is set to true
void asyncForceCustomApn | ( | dpyModem::result_handler_function | handler, |
const bool & | force = false , |
||
const dpyModem::AccessPointNetwork & | custom_apn = {} |
||
) |
- Parameters
-
handler callback function force flag that indicates if a manual selection is desired of not, true if desired , false if the APN is selected from the list (default) custom_apn the APN that wants to be set, in case force is set to true
void asyncForceNetworkMode | ( | const std::string & | modemid, |
dpyModem::NetworkMode | mode, | ||
dpyModem::result_handler_function | handler | ||
) |
- Parameters
-
modemid modem to which the operation will be requested mode network mode to be forced
- Remarks
- Select dpyModem::NetworkMode::MODE_ANY so that the best one is selected automatically (stop forcing a specific network mode)
- Parameters
-
handler
void asyncGetActiveSims | ( | const std::string & | modemid, |
dpyModem::sim_list_handler_function | handler | ||
) |
- Parameters
-
modemid modem to request the active sims from handler Handler to be called when a response comes from the service
- Remarks
- A sim is active/being used when the modem is trying to operate with it. The modem may have more than one sim card in the different sim slots but not all need to be enabled.
void asyncGetApnByImsi | ( | const std::string & | imsi, |
dpyModem::get_apn_by_imsi_handler_function | handler | ||
) |
- Parameters
-
imsi the value that will be checked handler callback function
- Remarks
- This method returns the APN from the list that will be used if a SIM card with the specified imsi is inserted. This does not mean that there is a specific APN for each imsi.
void asyncGetForcedAPN | ( | dpyModem::forced_apn_handler_function | handler | ) |
- Parameters
-
handler Callback function
void asyncGetInterfaceStatistics | ( | const std::string & | modemid, |
dpyModem::interface_statistics_function_handler | |||
) |
- Parameters
-
modemid modem to request the statistics info handler callback function
void asyncGetModemInfo | ( | const std::string & | modemid, |
dpyModem::modem_info_handler_function | handler | ||
) |
- Parameters
-
modemid modem to request the info from handler Handler to be called when a response comes from the service
void asyncGetModems | ( | dpyModem::modem_list_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetModemSims | ( | const std::string & | modemid, |
dpyModem::sim_list_handler_function | handler | ||
) |
- Parameters
-
modemid modem to request the available sims from handler Handler to be called when a response comes from the service
void asyncGetModemStatus | ( | const std::string & | modemid, |
dpyModem::modem_status_handler_function | handler | ||
) |
- Parameters
-
modemid modem to request the status from handler Handler to be called when a response comes from the service
void asyncGetSimInfo | ( | const std::string & | simid, |
dpyModem::sim_info_handler_function | handler | ||
) |
- Parameters
-
simid sim to request the info from handler Handler to be called when a response comes from the service
void asyncGetSimStatus | ( | const std::string & | simid, |
dpyModem::simStatus_handler_function | handler | ||
) |
- Parameters
-
simid sim to request the status from handler Handler to be called when a response comes from the service
void asyncIsDataConnectionEnabled | ( | const std::string & | modemid, |
dpyModem::modem_data_connection_status_handler_function | handler | ||
) |
- Parameters
-
modemid modem identifier handler Handler to be called when a response comes from the service
void asyncModifyApnByImsi | ( | const std::string & | current_imsi, |
const dpyModem::AccessPointNetwork & | new_apn, | ||
dpyModem::result_handler_function | handler | ||
) |
- Parameters
-
current_imsi the value of the imsi whose apn information will be changed new_apn the apn information structure that will substitute the current apn handler callback function
In order to understand the reason of the error code returned in the handler, please attend the following table:
ec (DPY_ERROR) | Result meaning |
---|---|
DPY_OK | The request was processed correctly |
DPY_NOT_FOUND | The APN could not be deleted |
DPY_NOT_PERMITTED | The APN to delete is being used at the moment and therefore it can't be deleted |
void asyncRequestModemOperation | ( | const std::string & | modemid, |
const dpyModem::ModemOperation & | operation, | ||
dpyModem::result_handler_function | handler | ||
) |
- Parameters
-
modemid modem to which the operation will be requested operation action to be performed by the modem handler Handler to be called when a new event comes from the service
void asyncSwitchCurrentSimCard | ( | const std::string & | modemid, |
dpyModem::result_handler_function | handler | ||
) |
- Remarks
- This is useful whenever the modem is dual-sim. Note that the Modem will try to work with the alternative sim slot , no matter if there is no sim.
- Warning
- This operation is persistent between reboots.
- Parameters
-
modemid modem identifier handler Handler to be called when a response comes from the service
void getCurrentSimSlot | ( | const std::string & | modemid, |
dpyModem::sim_slot_handler_function | handler | ||
) |
- Remarks
- This is useful whenever the modem is dual-sim.
- Parameters
-
modemid modem identifier handler Handler to be called when a response with the current sim slot has been received
void getModemListEvents_S | ( | dpyModem::modem_list_event_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a new event comes from the service
void getModemStatusEvents_S | ( | dpyModem::modem_status_handler_function | handler | ) |
- Parameters
-
handler callback function
void getModemWarningEvents_S | ( | dpyModem::modem_warning_event_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a new warning comes from the service
void getSimListEvents_S | ( | dpyModem::sim_list_event_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a sim list event comes from the service
void getSimStatusEvents_S | ( | dpyModem::simStatus_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a sim status event comes from the service
void getSimStatusEvents_U | ( | ) |
- Parameters
-
modemid modem to request the available sims from handler Handler to be called when a response comes from the service
bool isAlive | ( | ) |
- Returns
- Boolean indicating if Modem is alive
void monitorServiceAvailability_S | ( | dpyModem::service_availability_handler_function | handler | ) |
- Parameters
-
handler callback function