ModemClient Class Reference
Modem Client class that interacts with the modem Service.
#include <modemClient.h>
Inheritance diagram for ModemClient:
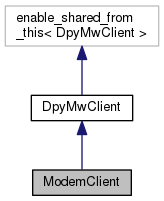
Public Types | |
enum | APN_OPERATION { ADD_APN, REMOVE_APN, CHANGE_APN } |
Types of APN operations as used in performApnOperation method. More... | |
Public Member Functions | |
ModemClient (std::string &ip, int dport, int sport) | |
void | getSmsNotifications_S (dpyModemPrivate::sms_notification_handler_function handler) |
void | getSmsNotifications_U () |
void | getApnByImsi (const std::string &imsi, dpyModem::get_apn_by_imsi_handler_function handler) |
void | performApnOperation (const APN_OPERATION &oper, const std::string &imsi, dpyModem::result_handler_function handler, const dpyModem::AccessPointNetwork &new_apn={}) |
void | asyncGetModems (dpyModem::modem_list_handler_function handler) |
void | asyncGetModemInfo (const std::string &modemid, dpyModem::modem_info_handler_function handler) |
void | asyncGetModemStatus (const std::string &modemid, dpyModem::modem_status_handler_function handler) |
void | getModemStatusEvents_S (dpyModem::modem_status_handler_function handler) |
void | getModemListEvents_S (dpyModem::modem_list_event_handler_function handler) |
void | getModemWarningEvents_S (dpyModem::modem_warning_event_handler_function handler) |
void | asyncRequestModemOperation (const std::string &modemid, const dpyModem::ModemOperation &operation, dpyModem::result_handler_function handler) |
void | asyncIsDataConnectionEnabled (const std::string &modemid, dpyModem::modem_data_connection_status_handler_function handler) |
void | asyncGetModemSims (const std::string &modemid, dpyModem::sim_list_handler_function handler) |
void | asyncGetActiveSims (const std::string &modemid, dpyModem::sim_list_handler_function handler) |
void | asyncSwitchCurrentSimCard (const std::string &modemid, dpyModem::result_handler_function handler) |
void | getCurrentSimSlot (const std::string &modemid, dpyModem::sim_slot_handler_function handler) |
void | getSimListEvents_S (dpyModem::sim_list_event_handler_function handler) |
void | getSimStatusEvents_S (dpyModem::simStatus_handler_function handler) |
void | getSimStatusEvents_U () |
void | asyncGetSimInfo (const std::string &simid, dpyModem::sim_info_handler_function handler) |
void | asyncGetSimStatus (const std::string &simid, dpyModem::simStatus_handler_function handler) |
void | asyncGetAllSmsInfo (const std::string &simid, dpyModemPrivate::get_sms_info_handler_function handler) |
void | getSmsEvents_S (dpyModemPrivate::sms_event_handler_function handler) |
void | asyncRequestSmsOperation (const std::string &simid, const dpyModemPrivate::SmsOperation &operation, const std::string &firstParameter, const std::string &secondParameter, dpyModem::result_handler_function handler) |
void | asyncGetCallSourceStatus (const std::string &simid, dpyModemPrivate::call_source_status_handler_function handler) |
void | getCallSourceUpdates_S (dpyModemPrivate::call_source_status_handler_function handler) |
void | getCallSourceUpdates_U () |
void | getVoiceCallEvents_S (dpyModemPrivate::voice_call_event_handler_function handler) |
void | getVoiceCallEvents_U () |
void | asyncRequestVoiceCallOperation (const std::string &simid, const dpyModemPrivate::VoiceCallOperation &operation, const std::string &firstParameter, dpyModem::result_handler_function handler) |
void | asyncGetLastVoiceCallInfo (const std::string &simid, dpyModemPrivate::get_sim_call_info_handler_function handler) |
void | asyncSetVoiceCallConfig (const std::string &modemid, const dpyModemPrivate::VoiceCallConfigParam ¶m, const std::string &value, dpyModem::result_handler_function handler) |
void | asyncGetVoiceCallConfig (const std::string &modemid, dpyModemPrivate::voice_call_config_handler_function handler) |
void | asyncForceCustomApn (const std::string &imsi, dpyModem::result_handler_function handler, const bool &force=false, const dpyModem::AccessPointNetwork &custom_apn={}) |
void | asyncForceCustomApn (dpyModem::result_handler_function handler, const bool &force=false, const dpyModem::AccessPointNetwork &custom_apn={}) |
void | asyncGetInterfaceStatistics (const std::string &modemid, dpyModem::interface_statistics_function_handler handler) |
void | asyncForceNetworkMode (const std::string &modemid, dpyModem::NetworkMode mode, dpyModem::result_handler_function handler) |
void | asyncGetForcedAPN (dpyModem::forced_apn_handler_function handler) |
![]() | |
DpyMwClient (std::string &ip, int dport, int sport) | |
void | start () |
void | stop () |
void | subscribe (int messageType, boost::any handler) |
void | unsubscribe (int messageType) |
bool | isAlive () |
void | monitorServiceAvailability_S (const f_hearbeat_handler &handler) |
void | monitorServiceAvailability_U () |
Additional Inherited Members | |
![]() | |
void | sendRequest (int requestType, int responseType, std::string content, boost::any handler, int ms_timeout=2000) |
![]() | |
map_proto_register_client | m_register_map |
std::mutex | m_map_mutex |
Member Enumeration Documentation
enum APN_OPERATION |