McuClient Class Reference
Class with the logic of the client.
#include <mcuClient.h>
Inheritance diagram for McuClient:
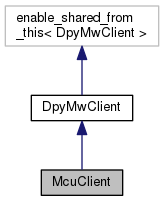
Public Member Functions | |
McuClient (std::string ip, int dport, int sport) | |
Constructor of the class. More... | |
~McuClient () | |
Destructor of the class. | |
void | asyncGetLedsConfig (dpyMcu::leds_handler_function handler) |
Method which requests the LED color state. More... | |
void | asyncSetLedsConfig (dpyMcu::leds_handler_function handler, dpyMcu::LedsColor color, std::uint32_t period, std::uint32_t duty_cycle) |
Method which requests to change the LED color state. More... | |
void | asyncSetPowerStateConfig (dpyMcu::power_states_handler_function handler, dpyMcu::PowerStates state, std::uint32_t flags) |
Method which requests to change Power State of OBU. More... | |
void | asyncSetBatteryProtectionConfig (dpyMcu::battery_protection_handler_function handler, float idle_voltage, float voltage_percentage_threshold, float hysteresis_percentage, std::uint32_t grace_period_minutes) |
Method which requests to set battery protection configuration values. More... | |
void | asyncGetBatteryProtectionConfig (dpyMcu::battery_protection_handler_function handler) |
Method which requests to get battery protection configuration values. More... | |
void | asyncSetRtcDateConfig (dpyMcu::rtc_date_handler_function handler, dpyMcu::DateConfig config) |
Method which requests to set RTC date configuration values. More... | |
void | asyncGetRtcDateConfig (dpyMcu::rtc_date_handler_function handler) |
Method which requests to get RTC date configuration values. More... | |
void | asyncSetTimersConfig (dpyMcu::timers_handler_function handler, std::uint32_t standby_minutes, std::uint32_t halt_minutes) |
Method which requests to change Standby and Halt Timers. More... | |
void | asyncGetTimersConfig (dpyMcu::timers_handler_function handler) |
Method which requests one message with Standby and Halt Timers. More... | |
void | asyncUpdateMCU (dpyMcu::update_firmware_handler_function handler) |
Method which updates the firmware of the MCU (the new image must be in the right PATH of the system) More... | |
void | asyncGetGUIDValues (dpyMcu::guid_handler_function handler) |
Method which requests one message with Part Number, Serial Number nad CIDL of the equipment. More... | |
void | asyncGetEQVar (dpyMcu::eqvar_handler_function handler) |
Method which requests equipment and variant identifiers. More... | |
void | asyncGetWorkingTimeValues (dpyMcu::working_time_handler_function handler) |
Method which requests one message with the Power-On, Standby and Halt States working half-hours. More... | |
void | asyncGetMCUStatus (dpyMcu::mcu_status_handler_function handler) |
Method which gets one message indicating hardware and software exceptions coming from MCU. More... | |
void | getPowerInformation_S (dpyMcu::power_mng_handler_function handler) |
Method which receives one message with Power information coming from the service periodically. More... | |
void | asyncGetPowerInformation (dpyMcu::power_mng_handler_function handler) |
Method which requests one message with Power information coming from the service. More... | |
void | asyncGetGpioValue (dpyMcu::gpio_handler_function handler, std::uint32_t gpio_number) |
Method which requests one message with GPIO information. More... | |
void | asyncGetGpiosEvents (dpyMcu::gpios_events_handler_function handler) |
Method which receives one message with Gpios events coming from the service. More... | |
void | asyncSetGpioValue (dpyMcu::gpio_handler_function handler, std::uint32_t gpio_number, bool gpio_value) |
Method which requests to set a GPIO pin with a value. More... | |
void | asyncGetI2CValue (dpyMcu::i2c_handler_function handler, unsigned char busid, unsigned char deviceid, std::list< unsigned char > address, int lenght) |
Method which requests one message with I2C information. More... | |
void | asyncSetI2CValue (dpyMcu::i2c_handler_function handler, unsigned char busid, unsigned char deviceid, std::list< unsigned char > address, std::list< unsigned char > values) |
Method which requests to set a I2C address pin with a value. More... | |
void | asyncGetVersion (dpyMcu::version_handler_function handler, dpyFirmware::Rfs rfs) |
Method which requests the firware version of the MCU. More... | |
void | asyncTestMisc (dpyMcu::test_misc_handler_function handler) |
Method which requests the current MISC status. More... | |
void | getPowerInformation_U () |
Method to stop receiving Power information coming from the service periodically. | |
void | asyncGetCanStatus (dpyMcu::can_status_handler_function handler) |
Method to get the current CAN Bus status. More... | |
void | asyncSetGpoDefValue (dpyMcu::gpio_handler_function handler, std::uint32_t gpo_number, bool gpo_value) |
Method to set default value to a GPO pin. More... | |
void | asyncGetGpoDefValue (dpyMcu::gpio_handler_function handler, std::uint32_t gpo_number) |
Method to get default value of a GPO pin. More... | |
void | asyncSetSerialPortProtocol (dpyMcu::serialport_handler_function handler, const std::string &portid, dpyMcu::SerialPortProtocol protocol) |
Method to set serial protocol to MCU-placed serial ports. More... | |
void | getCANMessages_S (dpyMcu::get_can_bus_message_handler_function handler) |
Establish the handler that the will be called when a CAN bus message is received. More... | |
void | getCANMessages_U () |
Stop the calls to the handler that updates CAN bus messages. | |
void | asyncEnablePowerButton (dpyMcu::set_power_button_state_handler_function handler) |
Method which enables the Power Button. More... | |
void | asyncDisablePowerButton (dpyMcu::set_power_button_state_handler_function handler) |
Method which enables the Power Button. More... | |
void | asyncGetPowerButtonState (dpyMcu::get_power_button_state_handler_function handler) |
Method which gets Power Button state. More... | |
void | asyncSetCanBusFilters (dpyMcu::result_handler_function handler, std::list< dpyMcu::CANBusFilter > &filter_list) |
Method to set CAN bus filters to MCU device. More... | |
void | asyncResetCanBusFilters (dpyMcu::result_handler_function handler) |
Method to reset CAN bus filters in MCU device. More... | |
void | asyncSetCanBusConfiguration (dpyMcu::result_handler_function handler, dpyMcu::CANBusConfiguration conf) |
Method to set CAN bus configuration to MCU device. More... | |
void | asyncResetMcu (dpyMcu::result_handler_function handler) |
Method to reset MCU device. More... | |
![]() | |
DpyMwClient (std::string &ip, int dport, int sport) | |
void | start () |
void | stop () |
void | subscribe (int messageType, boost::any handler) |
void | unsubscribe (int messageType) |
bool | isAlive () |
void | monitorServiceAvailability_S (const f_hearbeat_handler &handler) |
void | monitorServiceAvailability_U () |
Additional Inherited Members | |
![]() | |
void | sendRequest (int requestType, int responseType, std::string content, boost::any handler, int ms_timeout=2000) |
![]() | |
map_proto_register_client | m_register_map |
std::mutex | m_map_mutex |
Constructor & Destructor Documentation
McuClient | ( | std::string | ip, |
int | dport, | ||
int | sport | ||
) |
- Parameters
-
ip IP address to be binded to the client dport Port to use by dealer sport to use by subscriber
Member Function Documentation
void asyncDisablePowerButton | ( | dpyMcu::set_power_button_state_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncEnablePowerButton | ( | dpyMcu::set_power_button_state_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetBatteryProtectionConfig | ( | dpyMcu::battery_protection_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetCanStatus | ( | dpyMcu::can_status_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetEQVar | ( | dpyMcu::eqvar_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetGpiosEvents | ( | dpyMcu::gpios_events_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetGpioValue | ( | dpyMcu::gpio_handler_function | handler, |
std::uint32_t | gpio_number | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service gpio_number GPIO Number
void asyncGetGpoDefValue | ( | dpyMcu::gpio_handler_function | handler, |
std::uint32_t | gpo_number | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service gpo_number GPO number
void asyncGetGUIDValues | ( | dpyMcu::guid_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetI2CValue | ( | dpyMcu::i2c_handler_function | handler, |
unsigned char | busid, | ||
unsigned char | deviceid, | ||
std::list< unsigned char > | address, | ||
int | lenght | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service busid bus address deviceid device address address memory address lenght number of bytes to read
void asyncGetLedsConfig | ( | dpyMcu::leds_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetMCUStatus | ( | dpyMcu::mcu_status_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetPowerButtonState | ( | dpyMcu::get_power_button_state_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetPowerInformation | ( | dpyMcu::power_mng_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetRtcDateConfig | ( | dpyMcu::rtc_date_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetTimersConfig | ( | dpyMcu::timers_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncGetVersion | ( | dpyMcu::version_handler_function | handler, |
dpyFirmware::Rfs | rfs | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service rfs chosen to obtain the fw version installed (Possible values are "CURRENT" or "ALTERNATIVE")
void asyncGetWorkingTimeValues | ( | dpyMcu::working_time_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncResetCanBusFilters | ( | dpyMcu::result_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncResetMcu | ( | dpyMcu::result_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncSetBatteryProtectionConfig | ( | dpyMcu::battery_protection_handler_function | handler, |
float | idle_voltage, | ||
float | voltage_percentage_threshold, | ||
float | hysteresis_percentage, | ||
std::uint32_t | grace_period_minutes | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service idle_voltage idle voltage identified by ignition position off, engine off and stationary vehicle. Setting this value to 0 disables battery protection mode voltage_percentage_threshold voltage percentage calculated over idle voltage to set voltage battery protection mode threshold hysteresis_percentage hysteresis percentage calculated over idle voltage to enter and exit in battery protection mode grace_period_minutes grace period in minutes used to enter in halt mode irrevocably
- Remarks
- NOTE: With these values a low and a high threshold will be used to detect battery protection according next formula: – low_voltage_threshold = (idle_voltage * voltage_percentage / 100) - (idle_voltage * hysteresis_percentage / 100) – high_voltage_threshold = (idle_voltage * voltage_percentage / 100) + (idle_voltage * hysteresis_percentage / 100)
void asyncSetCanBusConfiguration | ( | dpyMcu::result_handler_function | handler, |
dpyMcu::CANBusConfiguration | conf | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service conf CAN bus configuration structure
void asyncSetCanBusFilters | ( | dpyMcu::result_handler_function | handler, |
std::list< dpyMcu::CANBusFilter > & | filter_list | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service filter_list List with CAN bus filter values
void asyncSetGpioValue | ( | dpyMcu::gpio_handler_function | handler, |
std::uint32_t | gpio_number, | ||
bool | gpio_value | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service gpio_number GPIO number gpio_value GPIO value
void asyncSetGpoDefValue | ( | dpyMcu::gpio_handler_function | handler, |
std::uint32_t | gpo_number, | ||
bool | gpo_value | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service gpo_number GPO number gpo_value GPO value
void asyncSetI2CValue | ( | dpyMcu::i2c_handler_function | handler, |
unsigned char | busid, | ||
unsigned char | deviceid, | ||
std::list< unsigned char > | address, | ||
std::list< unsigned char > | values | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service busid bus address deviceid device address address memory address. It's just ONE value. If you provide several values they will be concatenated to generate only ONE address. E.g. If you provide 0x10 0x20 0x30, it will produce a 0x102030 final address values values to be set
void asyncSetLedsConfig | ( | dpyMcu::leds_handler_function | handler, |
dpyMcu::LedsColor | color, | ||
std::uint32_t | period, | ||
std::uint32_t | duty_cycle | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service color to be set period periodic time to be set in seconds duty_cycle duty cycle to be set in percentage
void asyncSetPowerStateConfig | ( | dpyMcu::power_states_handler_function | handler, |
dpyMcu::PowerStates | state, | ||
std::uint32_t | flags | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service state State to be set flags Event flags to be set to exit from the indicated power state
void asyncSetRtcDateConfig | ( | dpyMcu::rtc_date_handler_function | handler, |
dpyMcu::DateConfig | config | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service config Date configuration struct
void asyncSetSerialPortProtocol | ( | dpyMcu::serialport_handler_function | handler, |
const std::string & | portid, | ||
dpyMcu::SerialPortProtocol | protocol | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service portid Serial Port identifier protocol Serial Port protocol
void asyncSetTimersConfig | ( | dpyMcu::timers_handler_function | handler, |
std::uint32_t | standby_minutes, | ||
std::uint32_t | halt_minutes | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service standby_minutes Minutes to Standby Timer halt_minutes Minutes to Halt Timer
void asyncTestMisc | ( | dpyMcu::test_misc_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void asyncUpdateMCU | ( | dpyMcu::update_firmware_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void getCANMessages_S | ( | dpyMcu::get_can_bus_message_handler_function | handler | ) |
- Parameters
-
handler callback function
void getPowerInformation_S | ( | dpyMcu::power_mng_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service