PrinterClient Class Reference
Class with the logic of the client.
#include <printerClient.h>
Inheritance diagram for PrinterClient:
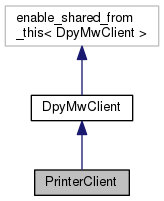
Public Member Functions | |
PrinterClient (std::string &ip, int dport, int sport) | |
Constructor of the class. More... | |
~PrinterClient () | |
Destructor of the class. | |
void | getPrinters (dpyPrinter::print_list_handler handler) |
Method which requests available printers. More... | |
void | getPrinterInfo (dpyPrinter::printer_info_handler handler, const std::string &printerid) |
Gets printer info. More... | |
void | addPrintJob (dpyPrinter::print_image_handler handler, const std::string &printerid, std::string image_path, std::list< PrintConfig > configs) |
Method which requests to print an image with a given configuration. More... | |
void | addPrintHtmlJob (dpyPrinter::print_html_handler handler, const std::string &printerid, std::string html_path, std::list< PrintConfig > configs) |
Method which requests to print an html with a given configuration. More... | |
void | getJobInfo (dpyPrinter::job_info_handler handler, int jobid) |
Gets job info. More... | |
void | getAllJobInfo (dpyPrinter::all_job_info_handler handler, const std::string &printerid) |
Gets all job info. More... | |
void | deleteJob (dpyPrinter::result_handler_function handler, int jobid) |
Deletes a job from the list. More... | |
void | setDefaultConfiguration (dpyPrinter::result_handler_function handler, const std::string &printerid, PrintConfig config) |
Method to set printer configuration. More... | |
void | getDefaultConfiguration (dpyPrinter::printerconfig_handler_function handler, const std::string &printerid) |
Gets printer config. More... | |
void | printTestTicket (dpyPrinter::result_handler_function handler, const std::string &printerid) |
Prints a test ticket. More... | |
void | storeNVImage (dpyPrinter::result_handler_function handler, const std::string &printerid, const std::string &image_path, int slotid) |
Store image in non-volatile memory. More... | |
void | deleteNVImage (dpyPrinter::result_handler_function handler, const std::string &printerid, int slotid) |
Delete image stored in non-volatile memory. More... | |
void | getNVImageList (dpyPrinter::printerimagelist_handler_function handler, const std::string &printerid) |
Gets list of images stored in non-volatile memory. More... | |
void | printNVImage (dpyPrinter::print_image_handler handler, const std::string &printerid, int slotId, std::list< PrintConfig > configs) |
Prints the image stored in the non-volatile memory area of the printer. More... | |
void | forcePrinterUpgrade (dpyPrinter::result_handler_function handler, const std::string &printerid) |
Forces upgrade of printer firmware. More... | |
void | printerListEvent_S (dpyPrinter::printer_list_event_handler_function handler) |
Subscribes to printer list events. More... | |
void | printerJobStatusEvent_S (dpyPrinter::job_status_event_handler_function handler) |
Subscribes to job status events. More... | |
void | printerEvent_S (dpyPrinter::printer_event_handler_function handler) |
Subscribes to printer events. More... | |
void | printerStatusEvent_S (dpyPrinter::printer_status_event_handler_function handler) |
Subscribes to printer status events. More... | |
void | paperLevelEvent_S (dpyPrinter::paper_level_event_handler_function handler) |
Subscribes to paper level events. More... | |
void | NVImageListEvent_S (dpyPrinter::printer_imagelist_event_handler_function handler) |
Subscribes to non-volatile memory images list events. More... | |
![]() | |
DpyMwClient (std::string &ip, int dport, int sport) | |
void | start () |
void | stop () |
void | subscribe (int messageType, boost::any handler) |
void | unsubscribe (int messageType) |
bool | isAlive () |
void | monitorServiceAvailability_S (const f_hearbeat_handler &handler) |
void | monitorServiceAvailability_U () |
Additional Inherited Members | |
![]() | |
void | sendRequest (int requestType, int responseType, std::string content, boost::any handler, int ms_timeout=2000) |
![]() | |
map_proto_register_client | m_register_map |
std::mutex | m_map_mutex |
Constructor & Destructor Documentation
PrinterClient | ( | std::string & | ip, |
int | dport, | ||
int | sport | ||
) |
- Parameters
-
ip IP address to be binded to the client dport Port to use by dealer sport to use by subscriber
Member Function Documentation
void addPrintHtmlJob | ( | dpyPrinter::print_html_handler | handler, |
const std::string & | printerid, | ||
std::string | html_path, | ||
std::list< PrintConfig > | configs | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service printerid printer id path Path and Name to the html to be printed configs list of configs for this specific job
void addPrintJob | ( | dpyPrinter::print_image_handler | handler, |
const std::string & | printerid, | ||
std::string | image_path, | ||
std::list< PrintConfig > | configs | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service printerid printer id image_path Path and Name to the image to be printed configs list of configs for this specific job
void deleteJob | ( | dpyPrinter::result_handler_function | handler, |
int | jobid | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service jobid job id
void deleteNVImage | ( | dpyPrinter::result_handler_function | handler, |
const std::string & | printerid, | ||
int | slotid | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service printerid printer id slotid Slot id where the image is stored (0 to 3)
void forcePrinterUpgrade | ( | dpyPrinter::result_handler_function | handler, |
const std::string & | printerid | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service printerid printer id
void getAllJobInfo | ( | dpyPrinter::all_job_info_handler | handler, |
const std::string & | printerid | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service printerid printer id for fetching jobs
void getDefaultConfiguration | ( | dpyPrinter::printerconfig_handler_function | handler, |
const std::string & | printerid | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service printerid printer id
void getJobInfo | ( | dpyPrinter::job_info_handler | handler, |
int | jobid | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service jobid job id
void getNVImageList | ( | dpyPrinter::printerimagelist_handler_function | handler, |
const std::string & | printerid | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service printerid printer id
void getPrinterInfo | ( | dpyPrinter::printer_info_handler | handler, |
const std::string & | printerid | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service printerid printer id
void getPrinters | ( | dpyPrinter::print_list_handler | handler | ) |
- Parameters
-
handler Handler to be called when a response comes from the service
void NVImageListEvent_S | ( | dpyPrinter::printer_imagelist_event_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a new NV memory images list event comes from the service
void paperLevelEvent_S | ( | dpyPrinter::paper_level_event_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a new printer status event comes from the service
void printerEvent_S | ( | dpyPrinter::printer_event_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a new event comes from the service
void printerJobStatusEvent_S | ( | dpyPrinter::job_status_event_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a new job status event comes from the service
void printerListEvent_S | ( | dpyPrinter::printer_list_event_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a new printer list event comes from the service
void printerStatusEvent_S | ( | dpyPrinter::printer_status_event_handler_function | handler | ) |
- Parameters
-
handler Handler to be called when a new printer status event comes from the service
void printNVImage | ( | dpyPrinter::print_image_handler | handler, |
const std::string & | printerid, | ||
int | slotId, | ||
std::list< PrintConfig > | configs | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service printerid printerid printer id slotid Slot id where the image is stored (0 to 3) configs list of configs for this specific job
void printTestTicket | ( | dpyPrinter::result_handler_function | handler, |
const std::string & | printerid | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service printerid printer id
void setDefaultConfiguration | ( | dpyPrinter::result_handler_function | handler, |
const std::string & | printerid, | ||
PrintConfig | config | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service printerid printer id config to modify in this printer
void storeNVImage | ( | dpyPrinter::result_handler_function | handler, |
const std::string & | printerid, | ||
const std::string & | image_path, | ||
int | slotid | ||
) |
- Parameters
-
handler Handler to be called when a response comes from the service printerid printer id image_path Path and name of the image to be saved slotid Slot id where the image will be stored (0 to 3)