Public Member Functions |
Public Attributes |
Protected Member Functions |
Properties |
List of all members
AudioManager Class Reference
Implementation of IAudioManager. Start point for interacting with Deepsy Audio service More...
Inheritance diagram for AudioManager:
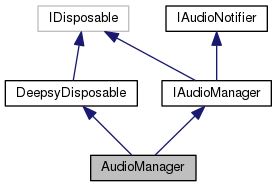
Public Member Functions | |
AudioManager (string ip) | |
AudioManager (AudioDealerConfiguration dealer, AudioSubscriberConfiguration subscriber) | |
AudioManager (int routePort, int publishPort) | |
IAudioConnection | CreateConnection (string Id, IAudioPort origin, IAudioPort destination) |
Create an audio connection between two ports More... | |
IAudioConnection | CreateConnection (string Id, IAudioPort origin, IAudioPort destination, int Priority) |
Create an audio connection between two ports More... | |
IAudioConnection | CreateConnection (string Id, IAudioPort origin, IAudioPort destination, int Priority, int volume, int gain) |
Create an audio connection between two ports More... | |
IAudioPort | CreatePort (string clientName, AudioPortType.Type type) |
Creates a user audio port More... | |
IEnumerable< IAudioConnection > | GetConnections () |
Returns the list of connections More... | |
IEnumerable< IAudioPort > | GetPorts () |
Return the list of available ports More... | |
IAudioTask | Play (IAudioPort port, string audioPath) |
IAudioTask | Record (IAudioPort port, string audioPath) |
void | RemoveConnection (IAudioConnection connection) |
Remove audio connection More... | |
void | RemovePort (IAudioPort port) |
Removes a port More... | |
void | StopTask (IAudioTask task) |
Stop task More... | |
IAudioTask | Play (IAudioPort port, string audioPath, IEnumerable< IAudioConfiguration > configurations) |
Play audio in a port using a specific configuration More... | |
IAudioTask | Record (IAudioPort port, string audioPath, IEnumerable< IAudioConfiguration > configurations) |
Record audio form a port to a file using specific configuration More... | |
IAudioTask | GetTaskStatus (int taskId) |
returns task information More... | |
IEnumerable< IAudioTask > | GetAllTaskInfo () |
returns all task information More... | |
IEnumerable< IAudioConfiguration > | GetConfiguration () |
Get Audio configurations More... | |
void | SetConfiguration (IAudioConfiguration configuration) |
Set default Audio configurations More... | |
void | SetPortGpio (IAudioPortGpio portGpio) |
Sets a GPO to be used as sink for audio port More... | |
void | UnsetPortGpio (IAudioPort port) |
Releases an audio port from GPO More... | |
IEnumerable< IAudioPortGpio > | GetPortGpio () |
Gets a IEnumerable with all GPO used as sinks for audio ports More... | |
override void | Dispose () |
Public Attributes | |
const int | MAX_PRIORITY_VALUE = 100 |
Max allowed value for connection priority. | |
const int | MIN_PRIORITY_VALUE = 0 |
Min allowed value for connection priority. | |
const int | DEFAULT_PRIORITY_VALUE = 0 |
Default value for connection priority if not defined. | |
const int | DEFAULT_VOLUME = -1 |
Default value for connection volume if not defined. | |
const int | DEFAULT_GAIN = -1 |
Default value for connection gain if not defined. | |
![]() | |
bool | IsDiposed => Disposed |
Protected Member Functions | |
override void | Dispose (bool disposing) |
Properties | |
EventHandler< IAudioPortListEvent > | PortListEvent |
EventHandler< IAudioConnectionListEvent > | ConnectionListEvent |
EventHandler< IAudioPortGpioEvent > | PortGpioEvent |
Additional Inherited Members | |
![]() | |
bool | Disposed = false |
![]() | |
EventHandler< IAudioPortListEvent > | PortListEvent |
Event raised by port changes More... | |
EventHandler< IAudioConnectionListEvent > | ConnectionListEvent |
Event raised by list of connection changes More... | |
EventHandler< IAudioPortGpioEvent > | PortGpioEvent |
Event raised by port gpio changes More... | |
Detailed Description
Member Function Documentation
IAudioConnection CreateConnection | ( | string | Id, |
IAudioPort | origin, | ||
IAudioPort | destination | ||
) |
- Parameters
-
Id Id of the new connection origin AudioPort origin of the new connection destination AudioPort destination of the new connection
- Returns
- Connection created
- Exceptions
-
NotAvailableException (NOT_AVAILABLE) Thrown when service is not available NotAvailableException (DPY_TIMEOUT) Thrown when service don't respond in time HalException Thrown when service returns an error
Implements IAudioManager.
IAudioConnection CreateConnection | ( | string | Id, |
IAudioPort | origin, | ||
IAudioPort | destination, | ||
int | Priority | ||
) |
- Parameters
-
Id Id of the new connection origin AudioPort origin of the new connection destination AudioPort destination of the new connection Priority Priority of the new connection
- Returns
- Connection created
- Exceptions
-
DeepsyHalException (NOT_AVAILABLE) Thrown when service is not available DeepsyHalException (DPY_TIMEOUT) Thrown when service don't respond in time DeepsyHalException Thrown when service returns an error
Implements IAudioManager.
IAudioConnection CreateConnection | ( | string | Id, |
IAudioPort | origin, | ||
IAudioPort | destination, | ||
int | Priority, | ||
int | volume, | ||
int | gain | ||
) |
- Parameters
-
Id Id of the new connection origin AudioPort origin of the new connection destination AudioPort destination of the new connection Priority Priority of the new connection volume Volume of the new connection gain Gain of the new connection
- Returns
- Connection created
- Exceptions
-
DeepsyHalException (NOT_AVAILABLE) Thrown when service is not available DeepsyHalException (DPY_TIMEOUT) Thrown when service don't respond in time DeepsyHalException Thrown when service returns an error
Implements IAudioManager.
IAudioPort CreatePort | ( | string | clientName, |
AudioPortType.Type | type | ||
) |
- Parameters
-
clientName client Name. It must start with user type Port type (input / ouput)
- Returns
- Port created
- Exceptions
-
NotAvailableException (NOT_AVAILABLE) Thrown when service is not available NotAvailableException (DPY_TIMEOUT) Thrown when service don't respond in time HalException Thrown when service returns an error
Implements IAudioManager.
IEnumerable<IAudioTask> GetAllTaskInfo | ( | ) |
- Returns
- List with task and its information
- Exceptions
-
NotAvailableException (NOT_AVAILABLE) Thrown when service is not available NotAvailableException (DPY_TIMEOUT) Thrown when service don't respond in time HalException Thrown when service returns an error
Implements IAudioManager.
IEnumerable<IAudioConfiguration> GetConfiguration | ( | ) |
- Returns
- List with default confiturations
- Exceptions
-
NotAvailableException (NOT_AVAILABLE) Thrown when service is not available NotAvailableException (DPY_TIMEOUT) Thrown when service don't respond in time HalException Thrown when service returns an error
Implements IAudioManager.
IEnumerable<IAudioConnection> GetConnections | ( | ) |
- Returns
- IConnection enumerable
- Exceptions
-
NotAvailableException (NOT_AVAILABLE) Thrown when service is not available NotAvailableException (DPY_TIMEOUT) Thrown when service don't respond in time HalException Thrown when service returns an error
Implements IAudioManager.
IEnumerable<IAudioPortGpio> GetPortGpio | ( | ) |
- Exceptions
-
DeepsyHalException (NOT_AVAILABLE) Thrown when service is not available DeepsyHalException (DPY_TIMEOUT) Thrown when service don't respond in time DeepsyHalException Thrown when service returns an error
Implements IAudioManager.
IEnumerable<IAudioPort> GetPorts | ( | ) |
- Returns
- IPort enumerable
- Exceptions
-
NotAvailableException (NOT_AVAILABLE) Thrown when service is not available NotAvailableException (DPY_TIMEOUT) Thrown when service don't respond in time HalException Thrown when service returns an error
Implements IAudioManager.
IAudioTask GetTaskStatus | ( | int | taskId | ) |
- Parameters
-
taskId Task ID
- Returns
- Task information
- Exceptions
-
NotAvailableException (NOT_AVAILABLE) Thrown when service is not available NotAvailableException (DPY_TIMEOUT) Thrown when service don't respond in time HalException Thrown when service returns an error
Implements IAudioManager.
IAudioTask Play | ( | IAudioPort | port, |
string | audioPath, | ||
IEnumerable< IAudioConfiguration > | configurations | ||
) |
- Parameters
-
port audioPath url of the file to play configurations configuration for the task
- Exceptions
-
NotAvailableException (NOT_AVAILABLE) Thrown when service is not available NotAvailableException (DPY_TIMEOUT) Thrown when service don't respond in time HalException Thrown when service returns an error
- Returns
Implements IAudioManager.
IAudioTask Record | ( | IAudioPort | port, |
string | audioPath, | ||
IEnumerable< IAudioConfiguration > | configurations | ||
) |
- Parameters
-
port audioPath
- Returns
- Exceptions
-
NotAvailableException (NOT_AVAILABLE) Thrown when service is not available NotAvailableException (DPY_TIMEOUT) Thrown when service don't respond in time HalException Thrown when service returns an error
Implements IAudioManager.
void RemoveConnection | ( | IAudioConnection | connection | ) |
- Parameters
-
connection Connection to be removed
- Exceptions
-
NotAvailableException (NOT_AVAILABLE) Thrown when service is not available NotAvailableException (DPY_TIMEOUT) Thrown when service don't respond in time HalException Thrown when service returns an error
Implements IAudioManager.
void RemovePort | ( | IAudioPort | port | ) |
- Parameters
-
port Port to be deleted
- Exceptions
-
NotAvailableException (NOT_AVAILABLE) Thrown when service is not available NotAvailableException (DPY_TIMEOUT) Thrown when service don't respond in time HalException Thrown when service returns an error
Implements IAudioManager.
void SetConfiguration | ( | IAudioConfiguration | configuration | ) |
- Parameters
-
configuration configuration to be set
- Returns
- List with default confiturations
- Exceptions
-
NotAvailableException (NOT_AVAILABLE) Thrown when service is not available NotAvailableException (DPY_TIMEOUT) Thrown when service don't respond in time HalException Thrown when service returns an error
Implements IAudioManager.
void SetPortGpio | ( | IAudioPortGpio | portGpio | ) |
- Parameters
-
portGpio AudioPortGpio port to set GPIO
- Exceptions
-
DeepsyHalException (NOT_AVAILABLE) Thrown when service is not available DeepsyHalException (DPY_TIMEOUT) Thrown when service don't respond in time DeepsyHalException Thrown when service returns an error
Implements IAudioManager.
void StopTask | ( | IAudioTask | task | ) |
- Parameters
-
task
- Exceptions
-
NotAvailableException (NOT_AVAILABLE) Thrown when service is not available NotAvailableException (DPY_TIMEOUT) Thrown when service don't respond in time HalException Thrown when service returns an error
Implements IAudioManager.
void UnsetPortGpio | ( | IAudioPort | port | ) |
- Parameters
-
port AudioPort port to to be released from GPO
- Exceptions
-
DeepsyHalException (NOT_AVAILABLE) Thrown when service is not available DeepsyHalException (DPY_TIMEOUT) Thrown when service don't respond in time DeepsyHalException Thrown when service returns an error
Implements IAudioManager.