AsyncSerialPort Class Reference
Class which manages the logic relative to send/receive messages from Asynchronous Serial Port. This class implements all the logic necessary to communicate with UART port.
#include <asyncSerialPort.h>
Inheritance diagram for AsyncSerialPort:
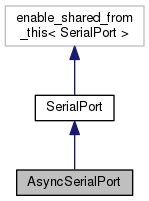
Public Member Functions | |
AsyncSerialPort (boost::asio::io_service &io, const char *port, int baud_rate, boost::asio::serial_port_base::flow_control::type flow, const F_READ &f=NULL, TypeRec type=nocanonical) | |
Constructor of the class. More... | |
AsyncSerialPort (boost::asio::io_service &io) | |
Constructor of the class. More... | |
~AsyncSerialPort () | |
Destructor of the class. | |
bool | open (boost::system::error_code &ec) |
Method to open serial port. More... | |
void | setReadCallback (const F_READ &f) |
Method to register the function that reads from the serial port. More... | |
bool | send (const char *buffer, int size) |
Method to send data to serial port asynchronously. More... | |
void | handle_send_to (const boost::system::error_code &error) |
Function handler to be called when an async sent took place. More... | |
void | handle_receive_from (const boost::system::error_code &error, size_t bytes_recvd) |
Function handler to be called when an async read took place. More... | |
![]() | |
SerialPort (boost::asio::io_service &io, const char *port, int baud_rate, boost::asio::serial_port_base::flow_control::type flow, TypeRec type=nocanonical) | |
Constructor of the class. More... | |
SerialPort (boost::asio::io_service &io) | |
Constructor of the class. More... | |
virtual | ~SerialPort () |
Destructor of the class. | |
bool | setPort (const char *port, int baud_rate, boost::asio::serial_port_base::flow_control::type flow) |
Method to establish serial port when it is not established in constructor. More... | |
const char * | getPort () const |
Method to take the serial port path. More... | |
bool | open (boost::system::error_code &ec) |
Method to open serial port. More... | |
bool | isOpened () const |
Method to query if serial port is opened by the current app. More... | |
bool | isBusy () const |
Method to query if serial port is opened by any app. More... | |
bool | isBusyOp () const |
Method to query if serial port is opened by any app. More... | |
void | close () |
Method to close serial port. | |
bool | flush () |
Method to clear the serial port buffer. More... | |
Protected Types | |
enum | { max_length = 1024 } |
typedef std::list< VC_DATA > | VC_PAQ |
![]() | |
typedef std::vector< char > | VC_DATA |
vector of characters | |
Protected Attributes | |
boost::asio::io_service::strand | m_strand |
F_READ | m_fRead |
Function handler to be called. | |
char | m_data [max_length] |
Data buffer. | |
VC_PAQ | m_vcDataSend |
Array with data buffer. | |
![]() | |
boost::asio::serial_port | m_serial_port |
Serial Port Object. | |
std::string | m_port |
Port Path name. | |
int | m_baud_rate |
Baud Rate to communicate. | |
boost::asio::serial_port_base::flow_control::type | m_flow_control |
UART flow control. | |
TypeRec | m_TypeR |
Canonical or non-canonical. | |
Additional Inherited Members | |
![]() | |
enum | TypeRec { canonical = 0, nocanonical = 1 } |
Enum to indicate how serial port must be configured. More... | |
Member Typedef Documentation
Constructor & Destructor Documentation
AsyncSerialPort | ( | boost::asio::io_service & | io, |
const char * | port, | ||
int | baud_rate, | ||
boost::asio::serial_port_base::flow_control::type | flow, | ||
const F_READ & | f = NULL , |
||
TypeRec | type = nocanonical |
||
) |
- Parameters
-
io io service port Path to the serial port baud_rate Rate of serial port to work flow UART flow control f handler to read async order (NULL default) type indicates the wat to open the serial port
|
explicit |
- Parameters
-
io io service
Member Function Documentation
void handle_receive_from | ( | const boost::system::error_code & | error, |
size_t | bytes_recvd | ||
) |
- Parameters
-
error error if it happened something incorrect bytes_recvd number of bytes read
void handle_send_to | ( | const boost::system::error_code & | error | ) |
- Parameters
-
error error if it happened something incorrect
bool open | ( | boost::system::error_code & | ec | ) |
- Parameters
-
ec error code
- Returns
- true if it is opened
|
virtual |
- Parameters
-
buffer Data buffer to be sent size Data length in bytes of the data buffer
- Returns
- true if serial port is opened and write took place
Implements SerialPort.
void setReadCallback | ( | const F_READ & | f | ) |
- Parameters
-
f the function that is called as a callback when new data from the port is read
Member Data Documentation
|
protected |
Strand to ensure thread safety